Genetic Algorithm
Genetic Algorithm
A real coded genetic algorithm for solving integer and mixed integer optimization problems, given by the following formulation:
Mathematical Formulation:
Minimize:
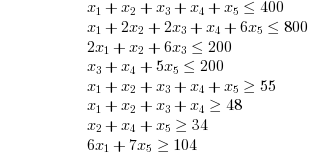
clc; // Number of decision variables nVar = 5; // Num\ber of constraints nCon = 8; //integer constraints intcon = 1:5; //coefficients of the linear term f = [-8 -2 -3 -1 -2]; // Hessian matrix H = [2 0 0 0 0; 0 2 0 0 0 0 0 6 0 0 0 0 0 8 0 0 0 0 0 4]; // Bounds of the problem lb = zeros(1,nVar); ub = 99*ones(1,nVar); // Constraint matrix A = [1 1 1 1 1; 1 2 2 1 6; 2 1 6 0 0; 0 0 1 1 5; -1 -1 -1 -1 -1 -1 -1 -1 -1 0; 0 -1 0 -1 -1; -6 0 0 0 -7]; b = [400 800 200 200 -55 -48 -34 -104]'; // Initial guess to the solver x0 = lb; // Calling the solver [xopt,fopt,exitflag,output] = intquadprog(H,f,intcon,A,b,[],[],lb,ub,x0) // Result representation if exitflag == 0 then disp("Optimal Solution Found") disp(xopt',"The optimal solution determined by the solver") disp(fopt,"The optimal objective function") elseif exitflag == 1 then disp("InFeasible Solution") else disp("Error encountered") end |
Expected Output:
Optimal Solution Found. The initial guess given to the solver 0. 0. 0. 0. 0. The optimal solution determined by the solver 16. 22. 5. 5. 7. The optimal objective function 807. |